Understanding Pointers in C
Pointers are a fundamental concept in the C programming language. They allow you to manipulate memory directly and are an essential tool for building efficient and flexible programs. In this article, we will explore what pointers are, how they work, and why they are so crucial in C programming.
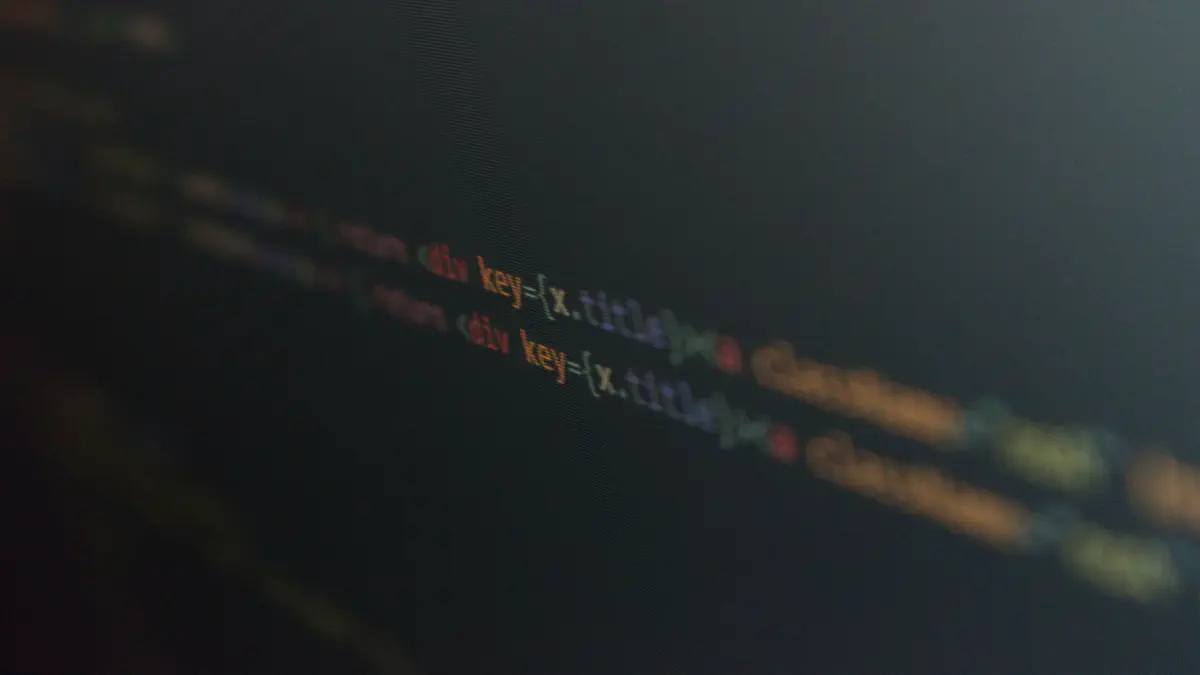
Overview
Pointers are a fundamental concept in the C programming language. They allow you to manipulate memory directly and are an essential tool for building efficient and flexible programs. In this article, we will explore what pointers are, how they work, and why they are so crucial in C programming.
What is a Pointer?
In C, a pointer is a variable that stores the memory address of another variable. Instead of holding the actual value of the data, a pointer points to the location in memory where the data is stored. This indirect referencing of data is what makes C so powerful and versatile.
Declaring Pointers
To declare a pointer variable in C, you use the *
symbol. Here’s an example:
int *ptr; // Declares a pointer to an integer
This declaration tells the compiler that ptr is a pointer to an integer. It doesn’t allocate memory for the integer; it merely reserves space to store the memory address of an integer.
Using Pointers
Once you have declared a pointer, you can use it to access and manipulate data indirectly. Here are some common operations involving pointers:
1. Assigning Values:
You can assign the address of a variable to a pointer using the address-of operator ```&```.
For example:
int num = 42;
int *ptr = # // ptr now points to the 'num' variable
2. Accessing Values:
To access the value stored at the memory location pointed to by a pointer, you use the dereference operator *
. For instance:
int value = *ptr; // 'value' now contains the value of 'num' (42)
3. Modifying Values
You can also use pointers to modify the value of the variable they point to:
*ptr = 100; // Changes the value of 'num' to 100
4. Pointer Arithmetic
In C, you can perform arithmetic operations on pointers. This is especially useful when working with arrays and dynamic memory allocation. For example:
int arr[5] = {1, 2, 3, 4, 5};
int *arrPtr = arr; // 'arrPtr' points to the first element of 'arr'
// Accessing elements using pointer arithmetic
int thirdElement = *(arrPtr + 2); // 'thirdElement' contains 3
Why Use Pointers?
Understanding pointers is essential for several reasons:
-
Dynamic Memory Allocation: Pointers are used to allocate and deallocate memory dynamically, enabling the creation of data structures like linked lists and trees.
-
Efficiency: Pointers allow you to work directly with memory, which can lead to more efficient code and better performance.
-
Passing by Reference: Pointers are used to pass variables to functions by reference, so changes made to the variable inside the function persist outside the function.
-
Interfacing with Hardware: In embedded systems and low-level programming, pointers are crucial for interfacing with hardware devices and memory-mapped registers.
Conclusion
Pointers are a powerful and essential feature of the C programming language. They allow you to work with memory addresses directly, providing flexibility, efficiency, and control over your programs. While they may seem complex at first, mastering pointers is a valuable skill for any C programmer. With practice and a solid understanding of their concepts, you’ll be well on your way to writing efficient and robust C code.